Adding custom events to calendar
The article describes how to add custom events for the IDelivery object type to the calendar. The object is used to register orders for delivery. The IDelivery object contains the following fields:
- Basic fields (Name, Date Created, Date Modified, Author, Change Creator);
- Dispatch date (date/time);
- Expected delivery date (date/time);
- Delivery address (text);
- Shipment delivered (Yes/No);
- Executor (user, connection type - single);
- Informed User (User, connection type - many-to-many).
There are two ways to add a custom event type to the calendar:
- In the Calendar section, choose the event time/date and click on it; a popup window will appear with a list of all available events types;
- In the Calendar section, click on the "Add" button; a list of all possible events will open.
Example of the data display
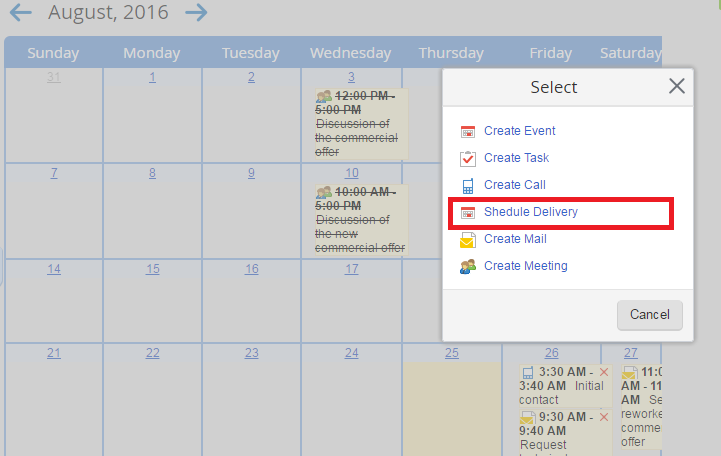
Fig. 1 Adding an event by opening a pop-up window
Fig. 2 Adding an event by clicking the Add button
Extension methods (interface)
The extension point (interface) IEventAddAction has the following methods:
/// <summary>
/// Button UID
/// </summary>
string Uid { get; }
/// <summary>
/// Name
/// </summary>
string Name { get; }
/// <summary>
/// Link to the form that adds an event
/// To define the start date and the end date of the event the following expressions can be added to the URL {0} – start date, {1} – end date
/// for example - /Event/Add?start={0}&end={1}
/// return urlHelper.Action("Add", "NameController", new { area = RouteProvider.AreaName, start = "{0}", end = "{1}" });
/// </summary>
string Url(RequestContext context);
/// <summary>
/// A parameter that opens URL in a modal window. If the parameter is null, the link opens in the parent window
/// for example - new { id = "meetingWindow",title = "Create meeting", width = 800, onOpenScript = "alert(’open’)"}
/// </summary>
dynamic WindowSettings { get; }
/// <summary>
/// Link to an action icon
/// </summary>
string Icon { get; }
/// <summary>
/// Display order
/// </summary>
int Order { get; }
/// <summary>
/// Whether to use this action for shared calendars
/// </summary>
bool AllowInShared { get; }
Example of the extension point class
[Component]
public class EventAddAction : IEventAddAction
{
public string Uid
{
get { return "add-calendar-delivery-event"; }
}
public string Name
{
get { return SR.T("Add an event "); }
}
public string Url(RequestContext context)
{
var urlHelper = new UrlHelper(context);
return urlHelper.Action("Add", "Catalogs", new
{
area = EleWise.ELMA.BPM.Web.Common.RouteProvider.AreaName,
uid = InterfaceActivator.UID<IDelivery>()
});
}
public dynamic WindowSettings
{
get { return null; }
}
public string Icon
{
get { return "#x16/entity.png"; }
}
public int Order
{
get { return 30; }
}
public bool AllowInShared
{
get { return false; }
}
}
Note
In the above code, the Add method is used to generate a link to the IDelivery object instance in the Catalogs controller. The object’s UID must be transmitted to the method as well.